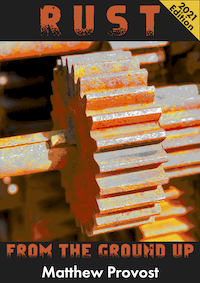
Rust From the Ground Up
The Rust CLI Programming book by Matthew Provost
New! Updated for the 2021 Edition of Rust and clap version 3
In 2024, developers voted for Rust as their most admired programming language for the second year in a row.
This book is for "day one" Rust programmers who want to achieve fluency in idiomatic Rust by implementing real-world programs, without fighting the borrow checker. Each example-heavy chapter implements a single CLI program in Rust based on the original BSD Unix source code. (No experience with programming in C required!).
"Rust From the Ground Up" flattens Rust's learning curve, teaching you how to build practical, real-world applications which handle realistic conditions such as edge cases and errors. Every chapter in Rust From the Ground Up takes a “show, don’t tell” approach: examining the original BSD sources for a well-known Unix CLI program, and introducing just enough Rust features to build a fully functional copy. After reading this book, you will have gained the confidence and intuition to write production-quality Rust programs.